Lecture 01: Course Introduction
Welcome to the Spring 2022 edition of COSC 211: Data Structures!!
Overview
- Motivation
- Course Structure and Expectations
- Course Overview
- First Task: The
List
ADT
-
List
Implementations
Motivating Example
3 Tasks
Task 1
Sort a list of numbers.
Task 2
Play chess well.
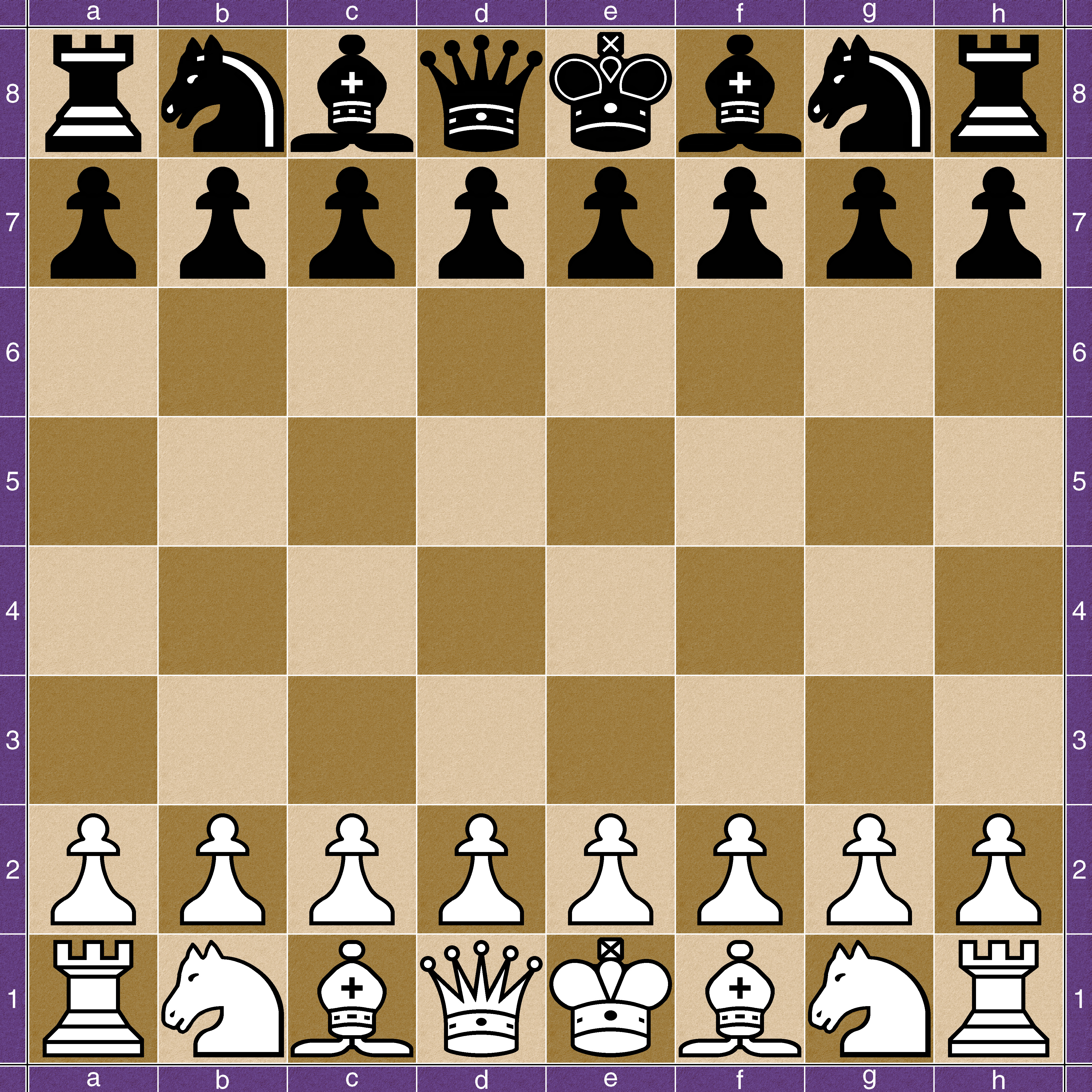
Task 3
Identify pictures of faces.
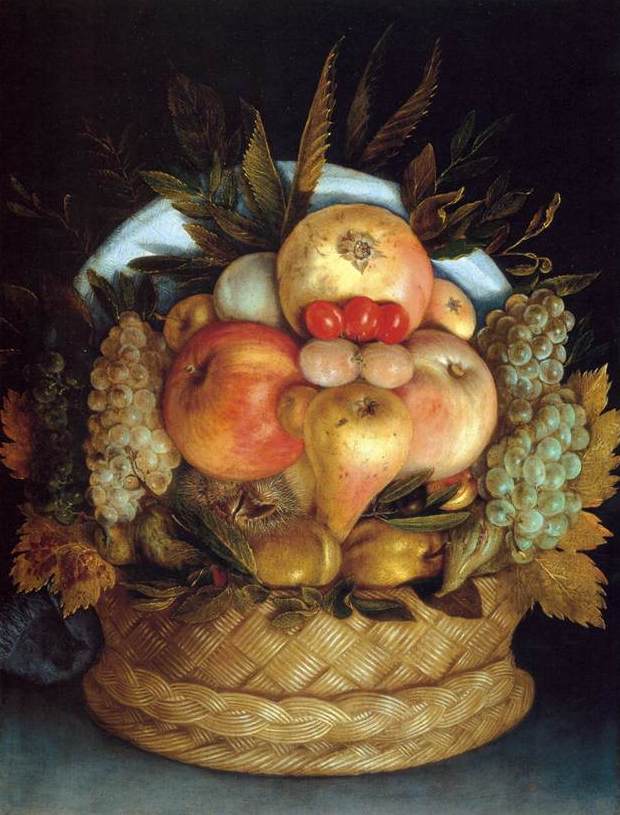
Questions
Consider the following three tasks:
- Sort a list of numbers
- Play chess well
- Identify pictures of faces
Question 1. Which tasks can computers effectively perform?
Question 2. In what ways are the tasks fundamentally different?
Which Tasks Can a Computer Do?
- Sort a list of numbers
- Play chess well
- Identify pictures of faces
How Are These Tasks Different?
- Sort a list of numbers
- Play chess well
- Identify pictures of faces
Nonetheless
Computers can detect faces well…
- e.g. Snapchat lenses
- face swap
… most of the time
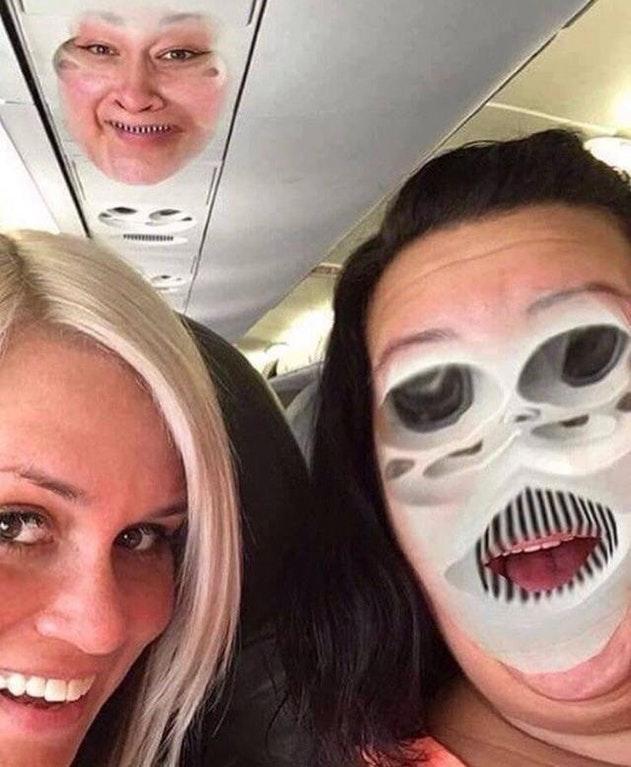
Treachery of Images I
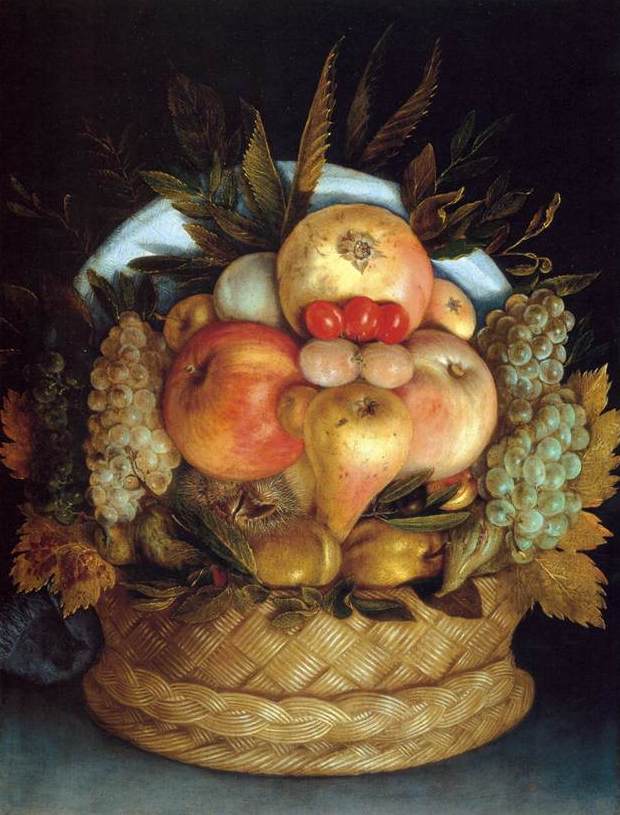
Treachery of Images III
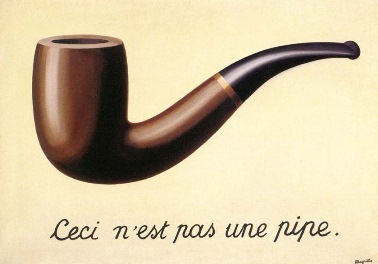
Situation with Chess
- best AI beats best humans at chess
- still unknown: can player 1 or player 2 always force a win
- known: at least one player can force a draw or win
Perfect chess play is possible in principle, not practical with current technology
- no known efficient procedure to generate “perfect” next move
Tasks, Revisited
- Sort a list of numbers
- solvable in practice
- formalizable: provable correctness and efficiency
- Play chess well
- solvable in practice
- formalizable, but formal solutions are impractical
- Identify pictures of faces
- solvable in practice
- not formalizable, can never guarantee correctness
Questions
How certain is “certain?”
- What tasks must a computer perform correctly & efficiently?
This Course
In this course we study “elementary” tasks and their solutions
- basic building blocks
- understand their correctness and efficiency from first principles
Why Study Data Structures?
- Power tools of programming
- Foundation for computing systems
- Generic solutions to common problems
- Understand efficiency
- Reason formally about tasks
Course Structure and Expectations
Meetings
- Twice Weekly, 80 minute meetings
- Guided discussion style
- part lecture
- part discussion
- questions always welcomed
- Lectures focus on conceptual material
- Assignments express material from lectures in code
Coursework
- Weekly homework assignments
- write and test code
- apply code to solve a problem
- answer conceptual questions
- Occasional quizzes (take-home)
- 3 Exams (take-home)
Homework collaboration
- On a conceptual level, yes!
- Do not share code solutions
Opportunities for Help
- Asking questions:
- during lecture
- my office hours
- TA office hours (drop-in evening sessions, by appointment)
- Talk to each other!
- Peer tutors
Programming
- All assignments in the Java programming language
- Course is not intended to teach basic programming
- Expectation: you are already comfortable with object oriented programming
- familiar w/ Java or ready to port knowledge of another language
Life Cycle of a Problem
- Formally specify task to be performed
- Devise a procedure to perform the task
- Reason about correctness and performance of the procedure
- Implement the procedure in code
- Test your implementation for correctness and performance
Levels of Abstraction
-
Abstract Data Type (ADT)
- formally specifies the problem to be solved
-
Data Structure (DS)
- way of representing the ADT
- “strategy” for doing task specified by ADT
-
Implementation
- code that represents the DS
-
Execution
- investigate what program actually does
Topics Covered
- Linear data structures and abstract data types (ADTs); program correctness and analysis
- tree-based data structures
- randomized data structures
- graphs, advanced data structures, and applications
Example
Task: on input n
, return 1
Program:
int mystery(long n) {
if (n <= 1) return 1;
if (n % 2 == 0) return mystery(n / 2);
return mystery(3 * n + 1);
}
Amazing fact: it is unknown whether mystery
performs the task!