Project 02: Substitution Cipher
Outline
- Substitution Ciphers
- Project Demo
- Getting Started
Secrecy
Since ancient times, people have relied on secret storing and transmission of sensitive information:
- Military secrets
- coordination
- battle plans
- Secret diary
- Secure payments (e.g., debit/credit card transactions)
- …
Setup
- start with cleartext message
- message that contains sensitive info
- want to generate a ciphertext
- obscures sensitive info in cleartext
- cleartext can be recovered from ciphertext
- but only if you know how!
-
key is secret that allows one to recover cleartext
- key might be e.g., password
Ciphers and Keys
A cipher is a method of generating ciphertext from cleartext and vice versa
More formally, a cipher has two methods:
encrypt : (cleartext, key) -> ciphertext
decrypt : (ciphertext, key) -> cleartext
We require
- if cleartext encrypted and decrypted with same key, get the same message back
We hope
- without key, it is hard recover cleartext from ciphertext
Caesar Cipher
Idea: map each letter to another letter
- use a single (cylic) shift $k$ (key)
Example: a cyclic shift of $k = +3$:
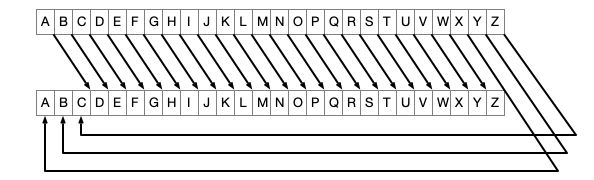
- to encrypt: follow arrow from each cleartext letter
- to decrypt: reverse direction
Encrypt Example
Encrypt RETREAT
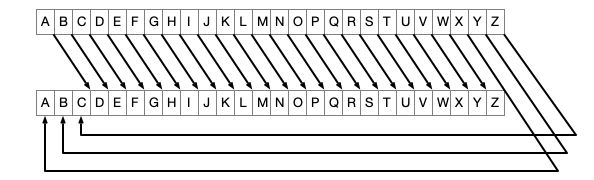
Decrypt Example
Decrypt DWWDFN
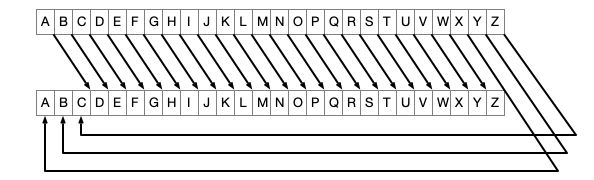
A More General Cipher
The substitution cipher
- choose an arbitrary one-to-one map between alphabets
- encryption/decryption the same as with Caesar
Substitution Example
Encrypt RETREAT
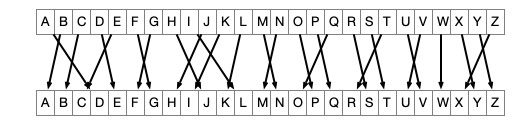
Project 02
Implement a substitution cipher!
Two differences with previous example:
- key should be a
long
value
- create
Random
object with long key
as seed
- use
Random
to create substitution table
- same
key
$\implies$ same table
- use table to encrypt or decrypt
- alphabet consists of all 255 possible
char
values
- not just chars
A
through Z
Today
- Download and run code
- Caesar cipher already implemented
- Use code to decrypt a message